Learn how to find an address or place with a Search component and the Geocoding service.
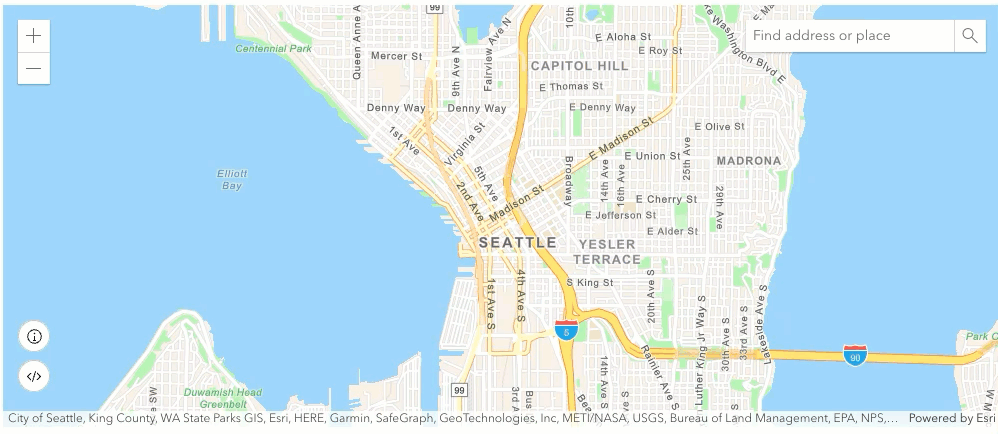
Geocoding is the process of converting address or place text into a location. The Geocoding service can search for an address or a place and perform reverse geocoding. Use the Search
component to access the Geocoding service and perform interactive searches.
In this tutorial, you will use the Search component to search for addresses and places.
Prerequisites
Steps
Create a new pen
- To get started, either complete the Display a map tutorial or .
Get an access token
You need an access token with the correct privileges to access the location services used in this tutorial.
- Go to the Create an API key tutorial and create an API key with the following privilege(s):
- Privileges
- Location services > Basemaps
- Location services > Geocoding
- Privileges
- In CodePen, set
esri
to your access token.Config.api Key Use dark colors for code blocks var esriConfig = { apiKey: "YOUR_ACCESS_TOKEN" };
To learn about other ways to get an access token, go to Types of authentication.
Update the map
A streets basemap layer is typically used in geocoding applications. Update the basemap
property to use the arcgis/navigation
basemap layer and change the position of the map to center on Seattle.
- Update the
basemap
attribute fromarcgis/topographic
toarcgis/navigation
. Then update thecenter
attribute to[-122.3321, 47.6062]
and set thezoom
attribute to12
to center on Seattle. .Use dark colors for code blocks <arcgis-map basemap="arcgis/navigation" center="-122.3321,47.6062" zoom="12"> <arcgis-zoom position="top-left"></arcgis-zoom> </arcgis-map>
Add Search component
The Search component is a control that allows you to interactively search for addresses and places. It provides suggestions as you type and allows you to select a result. When you select a result, it zooms to a location and displays a pop-up with the address information. By default, the component uses a locator
and source
that accesses the Geocoding service.
-
Inside the
<arcgis-map
component, add the> <arcgis-search
component and specify its position.> Use dark colors for code blocks <arcgis-map basemap="arcgis/navigation" center="-122.3321,47.6062" zoom="12"> <arcgis-zoom position="top-left"></arcgis-zoom> <arcgis-search position="top-right"></arcgis-search> </arcgis-map>
Run the app
In CodePen, run your code to display the map.
Try searching for the following locations:
Seattle
Space Needle
Hollywood Blvd
34.13419, -118.29636
The map should display a Search component that allows you to interactively search for addresses and places.
What's next?
Learn how to use additional API features and ArcGIS services in these tutorials: