What are time styles?
Time styles include all visualizations that involve date/time data. You can use these styles to visualize when and where an event occurs. Common examples involve the following:
- Timeline
- Before and after
- Age
- Geometry animation
- Attribute animation
Examples
Timeline
A timeline visualization displays date/time data along a continuous color ramp. It provides an immediate view at when phenomena occurred or when a value was recorded relative to all other data points.
The following example visualizes hurricane locations in the Atlantic Ocean recorded in the year 2000. This uses a color visual variable to show which hurricanes occurred early in the season versus late in the season.
visualVariables: [{
type: "color",
field: "Date_Time",
legendOptions: {
title: "Date of observation"
},
stops: [
{ value: new Date(2000, 7, 3).getTime(), color: "#00ffff"},
{ value: new Date(2000, 9, 22).getTime(), color: "#2f3f56"}
]
}]
Before and after
You can use a color visual variable with a diverging color ramp to visualize which events occurred before and after a specific date.
The following example visualizes clusters of hurricane locations summarized by whether they occurred before or after January 1, 1977 on average.
visualVariables: [{
type: "color",
field: "ISO_time",
legendOptions: {
title: "Before and after July 1"
},
stops: [
{ value: new Date(2013, 0, 1).getTime(), color: colors[0], label: "January 1" },
{ value: new Date(2013, 6, 1).getTime(), color: colors[1], label: "July 1" },
{ value: new Date(2013, 11, 31).getTime(), color: colors[2], label: "December 31" }
]
}]
Age
An age visualization shows the age of a feature (or the elapsed time up to a meaningful date or the current date) based on a date field. Age visualizations may be applied in the following scenarios:
- How long has an asset been in commission?
- How old is the incident report?
- How long has it been since the last inspection?
- Is an incident past due for resolution?
Age is typically defined using the DateDiff function in an Arcade expression and mapped with a color or size variable. It specifies a length of time with desired units.
valueExpression = "DateDiff( Now(), $feature.date_created, 'hours' )"
The following example visualizes clustered street condition complaints in New York City based on how much time passed before the incident was closed relative to its due date.
visualVariables: [{
type: "color",
valueExpression: "DateDiff($feature['closed_time'], $feature['due_time'], 'days')",
valueExpressionTitle: "Days it took to close incident",
stops: [
{ value: -21, color: colors[0], label: "21 weeks early" },
{ value: 0, color: colors[1], label: "On time" },
{ value: 21, color: colors[2], label: "21 weeks late" }
]
}]
Geometry animation
In most cases, time series animations involve assigning a layer a static renderer and filtering features over time. This is effective for features whose positions occur only at specific moments or windows of time (e.g. earthquakes, hurricanes, airplane travel). This requires a TimeSlider widget for control over the time window.
The layer in this example is rendered with a simple renderer and an animated gif showing a rotating hurricane symbol. This layer shows the current position of a hurricane relative to the slider thumb positions. Another layer is added to show the previous positions of the hurricanes relative to the time slider values.
const renderer = {
type: "simple",
label: "Observed hurricane location",
symbol: {
type: "picture-marker",
url: "/javascript/latest//assets/img/guide/visualization/demos/cyclone-marker.gif",
height: 20,
width: 20
},
visualVariables: [
{
type: "size",
field: "Category",
stops: [
{ value: 1, size: 12 },
{ value: 2, size: 16 },
{ value: 3, size: 20 },
{ value: 4, size: 24 },
{ value: 5, size: 28 }
]
}
]
};
Attribute animation
Attribute animations are animated visualizations showing how attributes in features fixed at unchanging locations change over time. These typically involve a time slider so users have the ability to explore moments of time or time windows.
For features that have a static position (such as sensors, buildings, countries, etc.), but that have attributes that vary over time (e.g. population, temperature, windspeed), you can dynamically update the renderer to reference data values tied to a specific moment in time, rather than filter features. This removes the need for downloading duplicate geometries to the client.
The renderer updates with every slider value change.
slider.on(["thumb-change", "thumb-drag"], (event) => {
updateYearDisplay(event.value);
updateRenderer(event.value);
updateHistogram(event.value);
});
function updateRenderer(value) {
const renderer = layer.renderer.clone();
const sizeVariable = renderer.visualVariables[0];
const colorVariable = renderer.visualVariables[1];
sizeVariable.valueExpression = getSizeValueExpression(value);
colorVariable.field = "F" + value;
renderer.visualVariables = [sizeVariable, colorVariable];
layer.renderer = renderer;
}
Related samples and resources
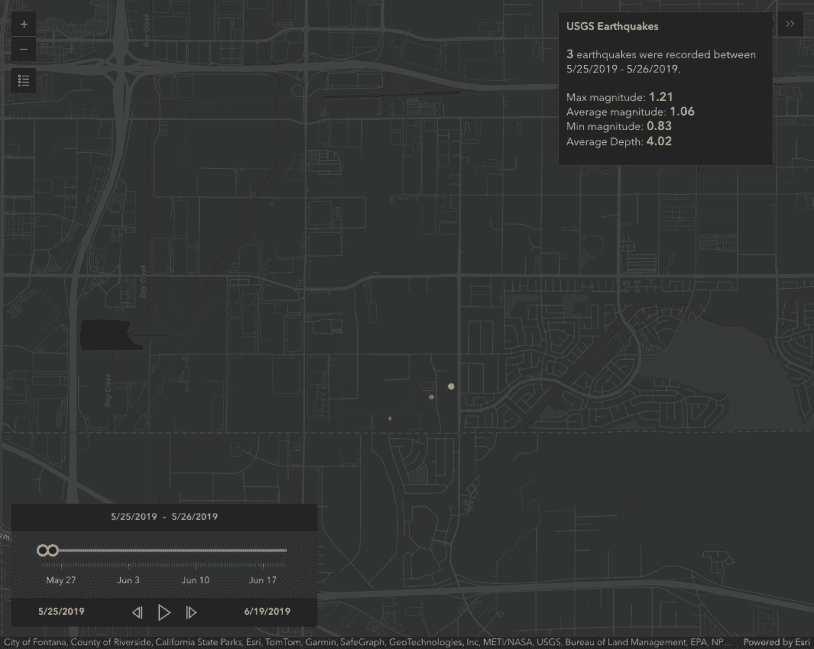
Filter features with TimeSlider
Filter features with TimeSlider
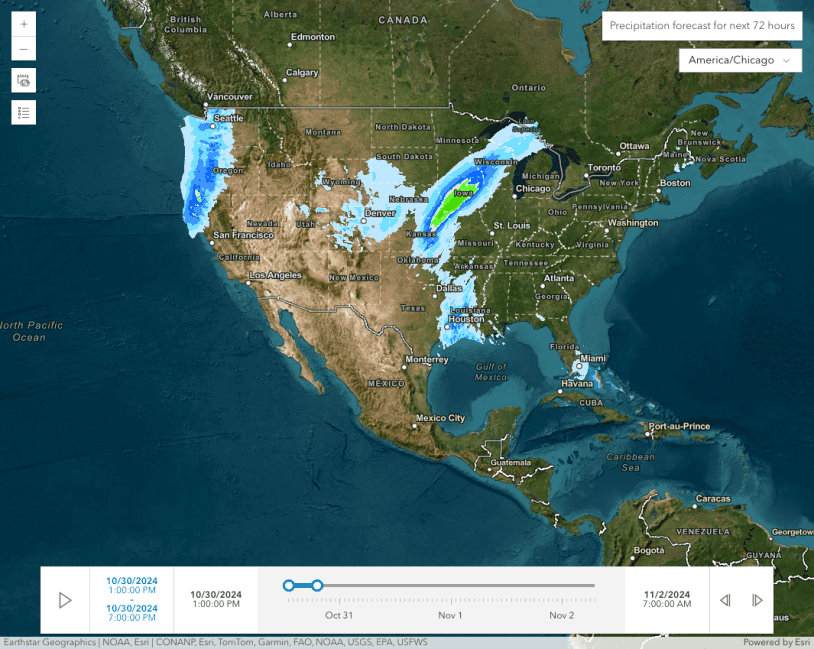
TimeSlider widget and time zone
TimeSlider widget and time zone
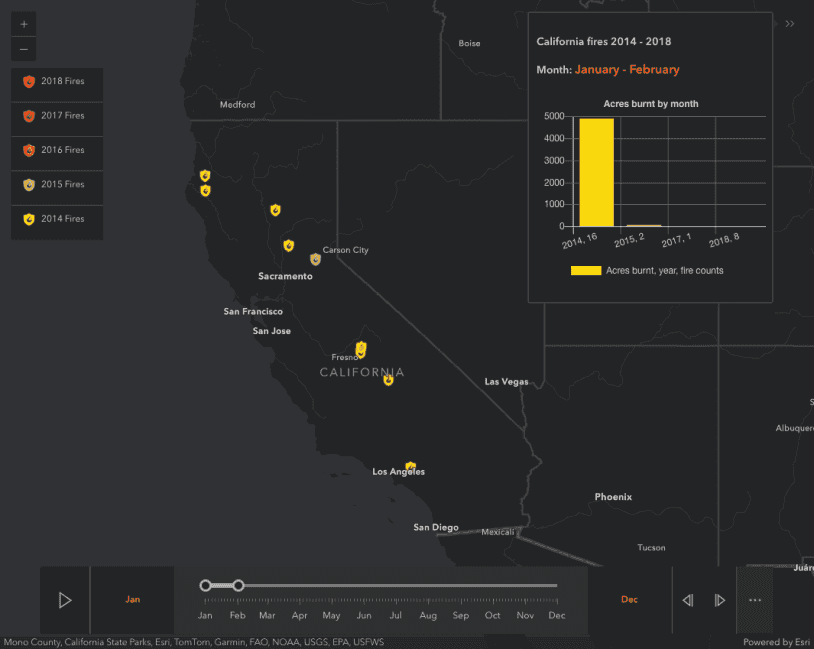
TimeSlider with timeOffset and actions
TimeSlider with timeOffset and actions
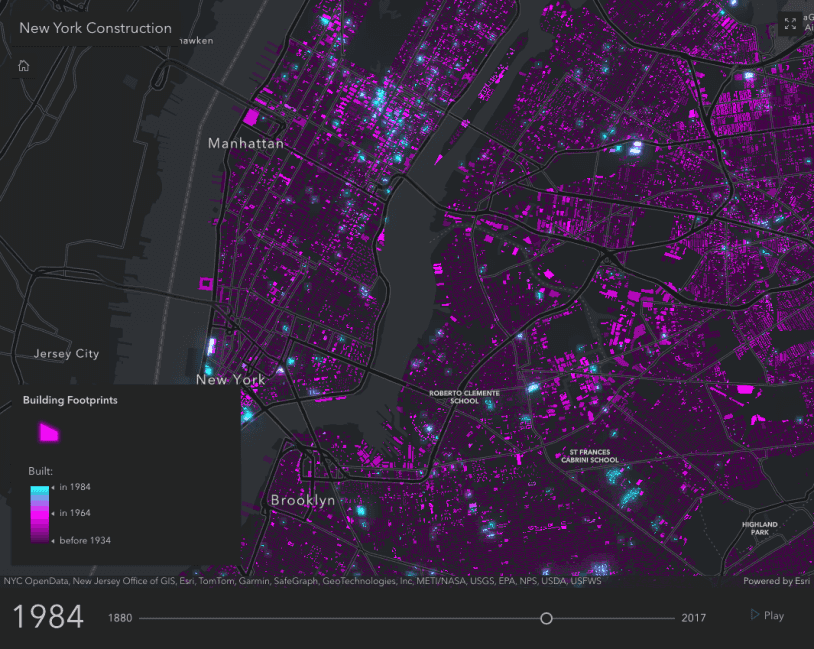
Animate color visual variable
Animate color visual variable
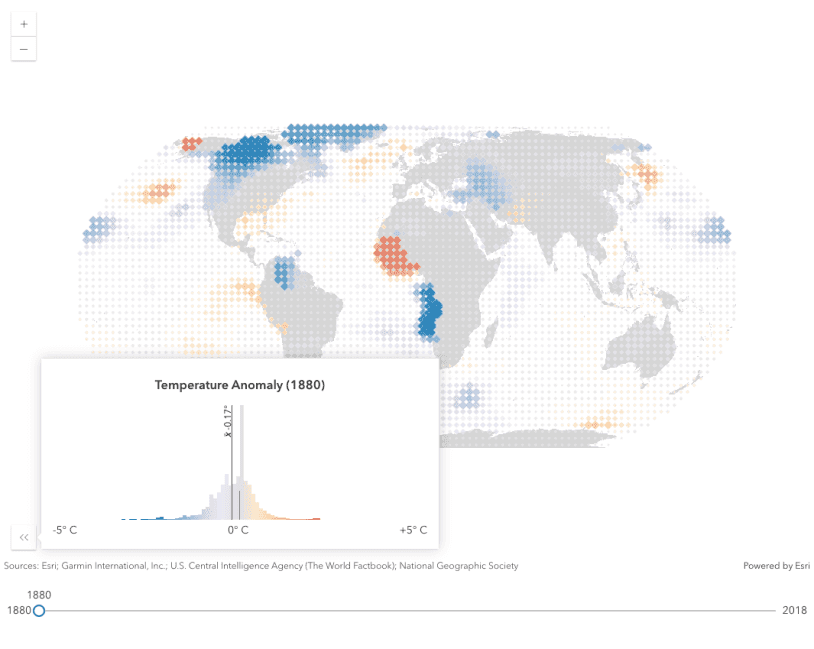
Update a renderer's attribute
Update a renderer's attribute's attribute
API support
2D | 3D | Arcade | Points | Lines | Polygons | Mesh | |
---|---|---|---|---|---|---|---|
Unique types | |||||||
Class breaks | |||||||
Visual variables | 1 | ||||||
Time | |||||||
Multivariate | |||||||
Predominance | |||||||
Dot density | |||||||
Charts | |||||||
Relationship | |||||||
Smart Mapping | 2 | 3 | 3 | 3 |
- 1. Color only
- 2. Size variable creators only supported for points
- 3. Size variable creators not supported in 3D