Learn how to display point, line, and polygon graphics in a map.
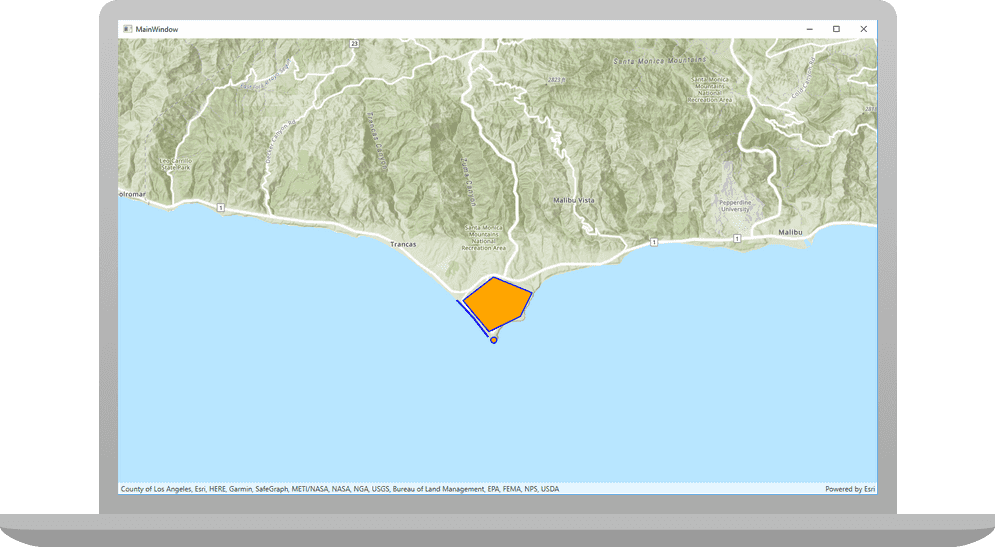
You typically use graphics to display geographic data that is not connected to a database and that is not persisted, like highlighting a route between two locations, displaying a search buffer around a point, or tracking the location of a vehicle in real-time. Graphics are composed of a geometry, symbol, and attributes.
In this tutorial, you display points, lines, and polygons on a map as graphics.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Ensure your development environment meets the system requirements.
Optionally, you may want to install the ArcGIS Maps SDK for .NET to get access to project templates in Visual Studio (Windows only) and offline copies of the NuGet packages.
Steps
Open a Visual Studio solution
-
To start the tutorial, complete the Display a map tutorial or download and unzip the solution.
-
Open the
.sln
file in Visual Studio. -
If you downloaded the solution, get an access token and set the API key.
An API Key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token using your ArcGIS Location Platform or ArcGIS Online account. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In Visual Studio, in the Solution Explorer, click App.xaml.cs.
-
In the App class, add an override for the
On
function to set theStartup() Api
property onKey ArcGISRuntimeEnvironment
.App.xaml.csUse dark colors for code blocks public partial class App : Application { protected override void OnStartup(StartupEventArgs e) { base.OnStartup(e); Esri.ArcGISRuntime.ArcGISRuntimeEnvironment.ApiKey = "YOUR_ACCESS_TOKEN"; } } }
-
If you are developing with Visual Studio for Windows, ArcGIS Maps SDK for .NET provides a set of project templates for each supported .NET platform. These templates follow the Model-View-ViewModel (MVVM) design pattern. Install the ArcGIS Maps SDK for .NET Visual Studio Extension to add the templates to Visual Studio (Windows only). See Install and set up for details.
Update the tutorial name used in the project (optional)
The Visual Studio solution, project, and the namespace for all classes currently use the name Display
. Follow the steps below if you prefer the name to reflect the current tutorial. These steps are not required, your code will still work if you keep the original name.
The tutorial instructions and code use the name Find
for the solution, project, and namespace. You can choose any name you like, but it should be the same for each of these.
-
Update the name for the solution and the project.
- In Visual Studio, in the Solution Explorer, right-click the solution name and choose Rename. Provide the new name for your solution.
- In the Solution Explorer, right-click the project name and choose Rename. Provide the new name for your project.
-
Rename the namespace used by classes in the project.
- In the Solution Explorer, expand the project node.
- Double-click MapViewModel.cs in the Solution Explorer to open the file.
- In the
Map
class, double-click the namespace name (View Model Display
) to select it, and then right-click and choose Rename....A Map - Provide the new name for the namespace.
- Click Apply in the Rename: DisplayAMap window that appears in the upper-right of the code window. This will rename the namespace throughout your project.
-
Build the project.
- Choose Build > Build solution (or press <F6>).
Add a graphics overlay
A graphics overlay is a container for graphics. It is used with a map view to display graphics on a map. You can add more than one graphics overlay to a map view. Graphics overlays are displayed on top of all the other layers.
-
In the Visual Studio > Solution Explorer, double-click MapViewModel.cs to open the file.
The project uses the Model-View-ViewModel (MVVM) design pattern to separate the application logic (view model) from the user interface (view).
Map
contains the view model class for the application, calledView Model.cs Map
. See the Microsoft documentation for more information about the Model-View-ViewModel pattern.View Model -
Add additional required
using
statements to yourMap
class.View Model Esri.ArcGISRuntime.UI
namespace contains theGraphic
class andEsri.ArcGISRuntime.Symbology
namespace contains the classes that define the symbols for displaying them.Use dark colors for code blocks using System; using System.Collections.Generic; using System.Text; using Esri.ArcGISRuntime.Geometry; using Esri.ArcGISRuntime.Mapping; using System.ComponentModel; using System.Runtime.CompilerServices; using Esri.ArcGISRuntime.Symbology; using Esri.ArcGISRuntime.UI;
-
In the view model, create a new property named
Graphics
. This will be a collection ofOverlays GraphicsOverlay
that will store point, line, and polygon graphics.Use dark colors for code blocks private Map? _map; public Map? Map { get { return _map; } set { _map = value; OnPropertyChanged(); } } private GraphicsOverlayCollection? _graphicsOverlays; public GraphicsOverlayCollection? GraphicsOverlays { get { return _graphicsOverlays; } set { _graphicsOverlays = value; OnPropertyChanged(); } }
-
Add a new method in the view model named
Create
. Add code to create a newGraphics GraphicsOverlay
and assign it to theGraphics
property.Overlays Use dark colors for code blocks private void SetupMap() { // Create a new map with a 'topographic vector' basemap. this.Map = new Map(BasemapStyle.ArcGISTopographic); } private void CreateGraphics() { // Create a new graphics overlay to contain a variety of graphics. var malibuGraphicsOverlay = new GraphicsOverlay(); // Add the overlay to a graphics overlay collection. GraphicsOverlayCollection overlays = new GraphicsOverlayCollection { malibuGraphicsOverlay }; // Set the view model's "GraphicsOverlays" property (will be consumed by the map view). this.GraphicsOverlays = overlays; }
-
In the
Map
constructor, add a call to theView Model Create
function.Graphics Use dark colors for code blocks public MapViewModel() { SetupMap(); CreateGraphics(); }
-
In the Visual Studio > Solution Explorer, double-click MainWindow.xaml to open the file.
-
Use data binding to bind the
Graphics
property of theOverlays Map
to theView Model MapView
control.Data binding and the Model-View-ViewModel (MVVM) design pattern allow you to separate the logic in your app (the view model) from the presentation layer (the view). Graphics can be added and removed from the graphics overlays in the view model and those changes appear in the map view through data binding.
Use dark colors for code blocks <esri:MapView x:Name="MainMapView" Map="{Binding Map, Source={StaticResource MapViewModel}}" GraphicsOverlays="{Binding GraphicsOverlays, Source={StaticResource MapViewModel}}" />
Add a point graphic
A point graphic is created using a map point and a marker symbol. A map point is defined with x and y coordinates and a spatial reference. For latitude and longitude coordinates, the spatial reference is WGS84.
-
In the Visual Studio > Solution Explorer, double-click MapViewModel.cs to open the file.
-
In the
Create
function, add code to create aGraphics MapPoint
and aSimpleMarkerSymbol
. To create theMapPoint
, provide longitude (x) and latitude (y) coordinates, and aSpatialReference
. Use theSpatialReferences.Wgs84
static property.Point graphics support a number of symbol types such as
SimpleMarkerSymbol
,PictureMarkerSymbol
andTextSymbol
. Learn more aboutSymbol
in the API Reference documentation.Use dark colors for code blocks // Set the view model's "GraphicsOverlays" property (will be consumed by the map view). this.GraphicsOverlays = overlays; // Create a point geometry. var dumeBeachPoint = new MapPoint(-118.8066, 34.0006, SpatialReferences.Wgs84); // Create a symbol to define how the point is displayed. var pointSymbol = new SimpleMarkerSymbol { Style = SimpleMarkerSymbolStyle.Circle, Color = System.Drawing.Color.Orange, Size = 10.0 }; // Add an outline to the symbol. pointSymbol.Outline = new SimpleLineSymbol { Style = SimpleLineSymbolStyle.Solid, Color = System.Drawing.Color.Blue, Width = 2.0 };
-
Create a
Graphic
with thepoint
andpoint
. Display theSymbol Graphic
by adding it to theGraphicsOverlay.Graphics
collection.Use dark colors for code blocks // Add an outline to the symbol. pointSymbol.Outline = new SimpleLineSymbol { Style = SimpleLineSymbolStyle.Solid, Color = System.Drawing.Color.Blue, Width = 2.0 }; // Create a point graphic with the geometry and symbol. var pointGraphic = new Graphic(dumeBeachPoint, pointSymbol); // Add the point graphic to graphics overlay. malibuGraphicsOverlay.Graphics.Add(pointGraphic);
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
If creating apps for Android or iOS, you will need the appropriate emulator, simulator, or device configured for testing (see System requirements for details)
You should see a point graphic in Point Dume State Beach.
Add a line graphic
A line graphic is created using a polyline and a line symbol. A polyline is defined as a sequence of points.
Polylines have one or more distinct parts, and each part is a sequence of points. For a continuous line, you can use the Polyline
constructor to create a polyline with just one part. To create a polyline with more than one part, use a PolylineBuilder
.
-
Create a
Polyline
and aSimpleLineSymbol
. To create thePolyline
, provide an array ofMapPoint
as the constructor argument.Line graphics support a number of symbol types such as
SimpleLineSymbol
andTextSymbol
. Learn more aboutSymbol
in the API Reference documentation.Use dark colors for code blocks // Add the point graphic to graphics overlay. malibuGraphicsOverlay.Graphics.Add(pointGraphic); // Create a list of points that define a polyline. List<MapPoint> linePoints = new List<MapPoint> { new MapPoint(-118.8215, 34.0140, SpatialReferences.Wgs84), new MapPoint(-118.8149, 34.0081, SpatialReferences.Wgs84), new MapPoint(-118.8089, 34.0017, SpatialReferences.Wgs84) }; // Create polyline geometry from the points. var westwardBeachPolyline = new Polyline(linePoints); // Create a symbol for displaying the line. var polylineSymbol = new SimpleLineSymbol(SimpleLineSymbolStyle.Solid, System.Drawing.Color.Blue, 3.0);
-
Create a
Graphic
with thepolyline
andpolyline
. Display theSymbol Graphic
by adding it to theGraphicsOverlay.Graphics
collection.Use dark colors for code blocks // Create a symbol for displaying the line. var polylineSymbol = new SimpleLineSymbol(SimpleLineSymbolStyle.Solid, System.Drawing.Color.Blue, 3.0); // Create a polyline graphic with geometry and symbol. var polylineGraphic = new Graphic(westwardBeachPolyline, polylineSymbol); // Add polyline to graphics overlay. malibuGraphicsOverlay.Graphics.Add(polylineGraphic);
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
You should see a point and line graphic along Westward Beach.
Add a polygon graphic
A polygon graphic is created using a polygon and a fill symbol. A polygon is defined as a sequence of points that describe a closed boundary.
Polygons have one or more distinct parts. Each part is a sequence of points that comprise a closed boundary. For a single area with no openings, you can use the Polygon
constructor to create a polygon made of just one part. To create a polygon that consists of more than one part, use a PolygonBuilder
.
-
Create a
Polygon
and aSimpleFillSymbol
. To create thePolygon
, provide an array ofMapPoint
s.Polygon graphics support a number of symbol types such as
SimpleFillSymbol
,PictureFillSymbol
,SimpleMarkerSymbol
andTextSymbol
. Learn more aboutSymbol
in the API Reference documentation.Use dark colors for code blocks // Add polyline to graphics overlay. malibuGraphicsOverlay.Graphics.Add(polylineGraphic); // Create a list of points that define a polygon boundary. List<MapPoint> polygonPoints = new List<MapPoint> { new MapPoint(-118.8190, 34.0138, SpatialReferences.Wgs84), new MapPoint(-118.8068, 34.0216, SpatialReferences.Wgs84), new MapPoint(-118.7914, 34.0164, SpatialReferences.Wgs84), new MapPoint(-118.7960, 34.0086, SpatialReferences.Wgs84), new MapPoint(-118.8086, 34.0035, SpatialReferences.Wgs84) }; // Create polygon geometry. var mahouRivieraPolygon = new Polygon(polygonPoints); // Create a fill symbol to display the polygon. var polygonSymbolOutline = new SimpleLineSymbol(SimpleLineSymbolStyle.Solid, System.Drawing.Color.Blue, 2.0); var polygonFillSymbol = new SimpleFillSymbol(SimpleFillSymbolStyle.Solid, System.Drawing.Color.Orange, polygonSymbolOutline);
-
Create an
Graphic
with thepolygon
andpolygon
. Display theSymbol Graphic
by adding it to theGraphicsOverlay.Graphics
collection.Use dark colors for code blocks // Create a fill symbol to display the polygon. var polygonSymbolOutline = new SimpleLineSymbol(SimpleLineSymbolStyle.Solid, System.Drawing.Color.Blue, 2.0); var polygonFillSymbol = new SimpleFillSymbol(SimpleFillSymbolStyle.Solid, System.Drawing.Color.Orange, polygonSymbolOutline); // Create a polygon graphic with the geometry and fill symbol. var polygonGraphic = new Graphic(mahouRivieraPolygon, polygonFillSymbol); // Add the polygon graphic to the graphics overlay. malibuGraphicsOverlay.Graphics.Add(polygonGraphic);
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
You should see a point, line, and polygon graphic around Mahou Riviera in the Santa Monica Mountains.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: