require(["esri/geometry/support/MeshMaterialMetallicRoughness"], (MeshMaterialMetallicRoughness) => { /* code goes here */ });
import MeshMaterialMetallicRoughness from "@arcgis/core/geometry/support/MeshMaterialMetallicRoughness.js";
esri/geometry/support/MeshMaterialMetallicRoughness
A material determines how a MeshComponent is visualized. This particular material (based on MeshMaterial) uses the metallic/roughness lighting model to enable physically based lighting. The metallic and roughness properties can be used to model various realistic materials including metals and plastics.
In this image you can see how metallic and roughness property values influence the display of the material:
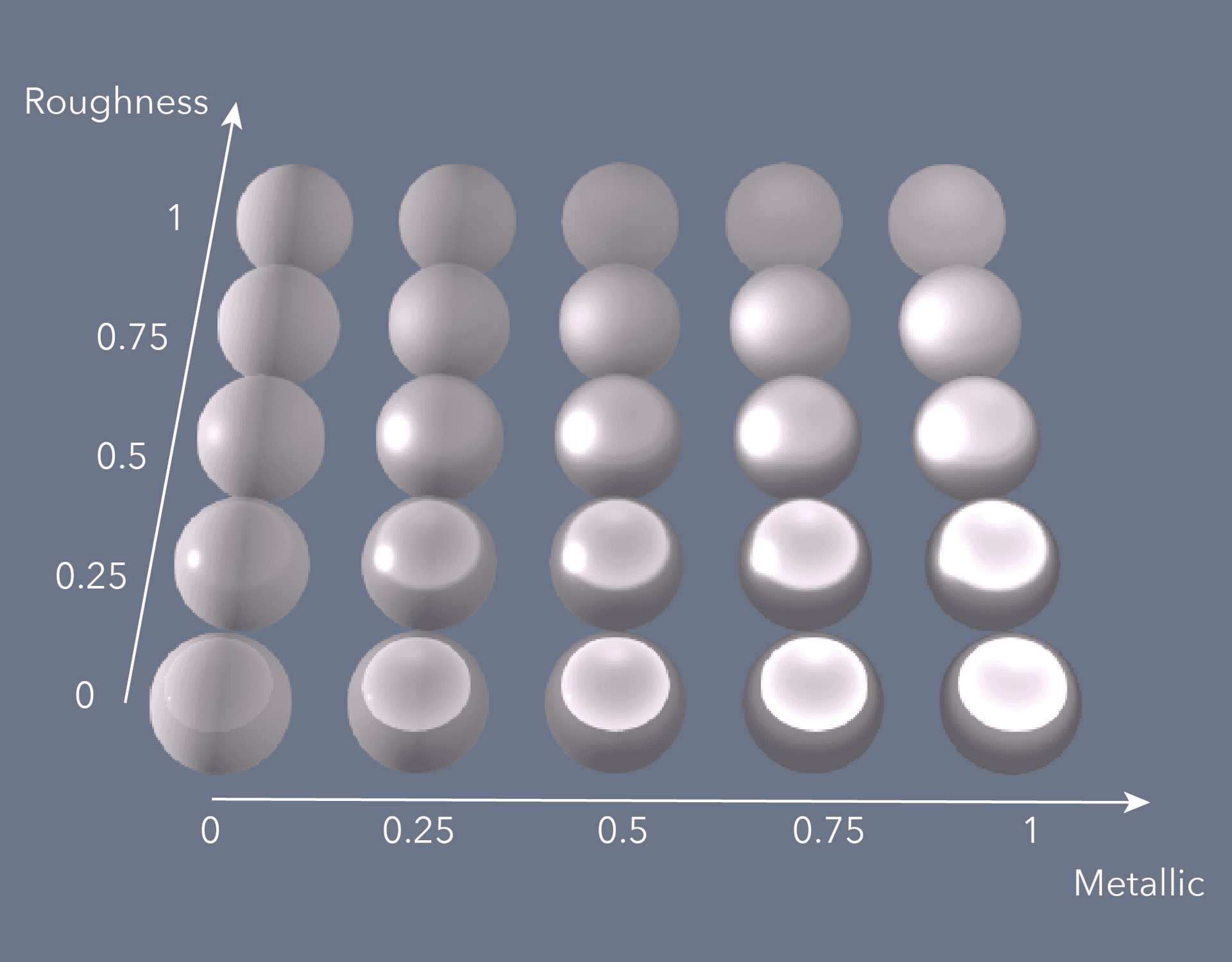
The metallicRoughnessTexture can be used to map specific metallic/roughness properties on different parts of the model.
Constructors
-
Parameterproperties Objectoptional
See the properties for a list of all the properties that may be passed into the constructor.
Property Overview
Name | Type | Summary | Class |
---|---|---|---|
Specifies how transparency on the object is handled. | MeshMaterial | ||
Specifies how transparency on the object is handled. | MeshMaterial | ||
Specifies a single, uniform color for the mesh component. | MeshMaterial | ||
Specifies a texture from which to get color information. | MeshMaterial | ||
A transformation of UV mesh coordinates used to sample the metallic roughness texture. | MeshMaterialMetallicRoughness | ||
The name of the class. | Accessor | ||
Specifies whether both sides of each triangle are displayed, or only the front sides. | MeshMaterial | ||
Specifies a single, uniform emissive color for the MeshComponent. | MeshMaterialMetallicRoughness | ||
Specifies a texture from which to get emissive color information. | MeshMaterialMetallicRoughness | ||
A transformation of UV mesh coordinates used to sample the emissive texture. | MeshMaterialMetallicRoughness | ||
Specifies how much the material behaves like a metal. | MeshMaterialMetallicRoughness | ||
Specifies a texture from which to get the combined metallic/roughness information. | MeshMaterialMetallicRoughness | ||
Specifies a texture from which to get normal information. | MeshMaterial | ||
A transformation of UV mesh coordinates used to sample the normal texture. | MeshMaterial | ||
Allows to specify a texture to get the occlusion information from. | MeshMaterialMetallicRoughness | ||
A transformation of UV mesh coordinates used to sample the occlusion texture. | MeshMaterialMetallicRoughness | ||
Indicates how rough the surface of the material is. | MeshMaterialMetallicRoughness |
Property Details
-
alphaCutoff
InheritedPropertyalphaCutoff Number
Inherited from MeshMaterial -
Specifies how transparency on the object is handled. If alphaMode is set to either
mask
orauto
this property specifies the cutoff value below which masking happens (that is, the corresponding part of the Mesh is rendered fully transparent).- Default Value:0.5
-
alphaMode
InheritedPropertyalphaMode String
Inherited from MeshMaterial -
Specifies how transparency on the object is handled. See also alphaCutoff.
Type Description opaque Alpha is ignored, and the object is rendered fully opaque. blend Alpha values are used for gradual transparency, blending between the object and its background. mask Alpha values are used for binary transparency, either displaying the object, or its background. See also alphaCutoff. auto The implementation mixes the mask
andblend
settings, masking belowalphaCutoff
and blending above it.Possible Values:"auto" |"blend" |"opaque" |"mask"
- Default Value:"auto"
-
Inherited from MeshMaterial
-
Specifies a single, uniform color for the mesh component. This can be autocast with a named string, hex string, array of rgb or rgba values, an object with
r
,g
,b
, anda
properties, or a Color object.
-
colorTexture
InheritedPropertycolorTexture MeshTextureautocast
Inherited from MeshMaterialAutocasts from Object|HTMLImageElement|HTMLCanvasElement|HTMLVideoElement|ImageData|String -
Specifies a texture from which to get color information. The texture is accessed using the uv coordinate specified for each vertex in the Mesh.vertexAttributes.
-
colorTextureTransform
colorTextureTransform MeshTextureTransform
Since: ArcGIS Maps SDK for JavaScript 4.27MeshMaterialMetallicRoughness since 4.15, colorTextureTransform added at 4.27. -
A transformation of UV mesh coordinates used to sample the metallic roughness texture. Texture transformations can be used to optimize re-use of textures, for example by packing many images into a single texture "atlas" and using the texture transform offset and scale to sample a specific region within this larger texture.
- Default Value:undefined
-
doubleSided
InheritedPropertydoubleSided Boolean
Inherited from MeshMaterial -
Specifies whether both sides of each triangle are displayed, or only the front sides.
- Default Value:true
-
Specifies a single, uniform emissive color for the MeshComponent. The emissiveColor is added to the base color of the component. This can be autocast with a named string, hex string, array of rgb values, an object with
r
,g
,b
properties, or a Color object. Note that the alpha channel is ignored for emissive colors.
-
emissiveTexture
emissiveTexture MeshTextureautocast
Autocasts from Object|HTMLImageElement|HTMLVideoElement|HTMLCanvasElement|ImageData|String -
Specifies a texture from which to get emissive color information. The texture is accessed using the uv coordinate specified for each vertex in the mesh vertex attributes. The colors in the texture are added to the base color of the component. When using both an
emissiveTexture
and an emissiveColor their values are multiplied and then added to the base color of the component.
-
emissiveTextureTransform
emissiveTextureTransform MeshTextureTransform
Since: ArcGIS Maps SDK for JavaScript 4.27MeshMaterialMetallicRoughness since 4.15, emissiveTextureTransform added at 4.27. -
A transformation of UV mesh coordinates used to sample the emissive texture. Texture transformations can be used to optimize re-use of textures, for example by packing many images into a single texture "atlas" and using the texture transform offset and scale to sample a specific region within this larger texture.
- Default Value:undefined
-
metallic
metallic Number
-
Specifies how much the material behaves like a metal. Values must be in the range 0 (non metal material) to 1 (metal material). Physically accurate materials are usually either a metal (1) or a non-metal (0) and not something inbetween.
- Default Value:1
-
metallicRoughnessTexture
metallicRoughnessTexture MeshTextureautocast
Autocasts from Object|HTMLImageElement|HTMLCanvasElement|HTMLVideoElement|ImageData|String -
Specifies a texture from which to get the combined metallic/roughness information. The metallic value should be stored in the
blue
channel, while the roughness value should be stored in thegreen
channel. Thered
andalpha
channels are ignored.The texture is accessed using the uv coordinate specified for each vertex in the mesh vertex attributes.
-
normalTexture
InheritedPropertynormalTexture MeshTextureautocast
Inherited from MeshMaterialAutocasts from Object|HTMLImageElement|HTMLVideoElement|HTMLCanvasElement|ImageData|String -
Specifies a texture from which to get normal information. The texture is accessed using the uv coordinate specified for each vertex in the Mesh.vertexAttributes.
-
normalTextureTransform
InheritedPropertynormalTextureTransform MeshTextureTransform
Inherited from MeshMaterialSince: ArcGIS Maps SDK for JavaScript 4.27MeshMaterial since 4.11, normalTextureTransform added at 4.27. -
A transformation of UV mesh coordinates used to sample the normal texture. Texture transformations can be used to optimize re-use of textures, for example by packing many images into a single texture "atlas" and using the texture transform offset and scale to sample a specific region within this larger texture.
- Default Value:undefined
-
occlusionTexture
occlusionTexture MeshTextureautocast
Autocasts from Object|HTMLImageElement|HTMLVideoElement|HTMLCanvasElement|ImageData|String -
Allows to specify a texture to get the occlusion information from. This can be used to simulate the effect of ambient light on the object. The texture is accessed using the uv coordinate specified for each vertex in the mesh vertex attributes. The occlusion value should be encoded in the red channel of the texture.
-
occlusionTextureTransform
occlusionTextureTransform MeshTextureTransform
Since: ArcGIS Maps SDK for JavaScript 4.27MeshMaterialMetallicRoughness since 4.15, occlusionTextureTransform added at 4.27. -
A transformation of UV mesh coordinates used to sample the occlusion texture. Texture transformations can be used to optimize re-use of textures, for example by packing many images into a single texture "atlas" and using the texture transform offset and scale to sample a specific region within this larger texture.
- Default Value:undefined
-
roughness
roughness Number
-
Indicates how rough the surface of the material is. Values must be in the range 0 (fully smooth surface) to 1 (fully diffuse surface).
- Default Value:1
Method Overview
Name | Return Type | Summary | Class |
---|---|---|---|
Adds one or more handles which are to be tied to the lifecycle of the object. | Accessor | ||
Returns true if a named group of handles exist. | Accessor | ||
Removes a group of handles owned by the object. | Accessor |
Method Details
-
Inherited from Accessor
Since: ArcGIS Maps SDK for JavaScript 4.25Accessor since 4.0, addHandles added at 4.25. -
Adds one or more handles which are to be tied to the lifecycle of the object. The handles will be removed when the object is destroyed.
// Manually manage handles const handle = reactiveUtils.when( () => !view.updating, () => { wkidSelect.disabled = false; }, { once: true } ); this.addHandles(handle); // Destroy the object this.destroy();
ParametershandleOrHandles WatchHandle|WatchHandle[]Handles marked for removal once the object is destroyed.
groupKey *optionalKey identifying the group to which the handles should be added. All the handles in the group can later be removed with Accessor.removeHandles(). If no key is provided the handles are added to a default group.
-
hasHandles
InheritedMethodhasHandles(groupKey){Boolean}
Inherited from AccessorSince: ArcGIS Maps SDK for JavaScript 4.25Accessor since 4.0, hasHandles added at 4.25. -
Returns true if a named group of handles exist.
ParametergroupKey *optionalA group key.
ReturnsType Description Boolean Returns true
if a named group of handles exist.Example// Remove a named group of handles if they exist. if (obj.hasHandles("watch-view-updates")) { obj.removeHandles("watch-view-updates"); }
-
Inherited from Accessor
Since: ArcGIS Maps SDK for JavaScript 4.25Accessor since 4.0, removeHandles added at 4.25. -
Removes a group of handles owned by the object.
ParametergroupKey *optionalA group key or an array or collection of group keys to remove.
Exampleobj.removeHandles(); // removes handles from default group obj.removeHandles("handle-group"); obj.removeHandles("other-handle-group");