What is a heatmap?
A Heatmap renders point features as a raster surface, emphasizing areas with a higher density of points along a continuous color ramp.
Why are heatmaps useful?
Heatmaps can be used as an alternative to clustering, opacity, and bloom to visualize overlapping point features. Unlike these alternative techniques for visualizing density, you can use a heatmap to weight the density of points based on a data value. This may reveal a different pattern than density calculated using location alone.
Location-only heatmap | Data-weighted heatmap |
---|---|
![]() |
![]() |
How a heatmap works
Heatmaps are created by setting a HeatmapRenderer instance to the renderer
property of the layer. The HeatmapRenderer has several key properties. The radius
determines the area of influence surrounding a point. For example, if you set a radius of 10 pixels, each pixel within 10 pixels of a single point will be assigned a density value. The pixel directly on top of the point will have the highest density. Smaller density values are assigned to pixels surrounding the point inversely proportional to the distance from the point. Pixels on the screen that fall outside that 10-pixel radius of all points will have a density value of 0
.
The density value of each pixel accumulates based on its proximity to multiple points. For example, a pixel within 10px of 10 points, will have a higher density value than pixels within 10x of only one point.
The color
in the renderer determine how to colorize pixels based on the ratio of a pixel's density value to the max
(i.e. pixel density / max
). Pixels with a density value at or above the max
have a density ratio of 1 and should be assigned the brightest color since these represent the hottest areas. The larger the max
, the fewer hot spots will exist in the map.
Examples
Location heatmap
To create a heatmap, you must apply a HeatmapRenderer to a point layer. Set the renderer properties using the guidelines outlined above. Open this example in CodePen and adjust the radius
and max
to see how these properties impact the heatmap.
const colors = ["rgba(115, 0, 115, 0)", "#820082", "#910091", "#a000a0", "#af00af", "#c300c3", "#d700d7", "#eb00eb", "#ff00ff", "#ff58a0", "#ff896b", "#ffb935", "#ffea00"];
layer.renderer = {
type: "heatmap",
colorStops: [
{ color: colors[0], ratio: 0 },
{ color: colors[1], ratio: 0.083 },
{ color: colors[2], ratio: 0.166 },
{ color: colors[3], ratio: 0.249 },
{ color: colors[4], ratio: 0.332 },
{ color: colors[5], ratio: 0.415 },
{ color: colors[6], ratio: 0.498 },
{ color: colors[7], ratio: 0.581 },
{ color: colors[8], ratio: 0.664 },
{ color: colors[9], ratio: 0.747 },
{ color: colors[10], ratio: 0.83 },
{ color: colors[11], ratio: 0.913 },
{ color: colors[12], ratio: 1 }
],
radius: 18,
maxDensity: 0.04625,
minDensity: 0
};
Data-weighted heatmap
This example modifies the previous heatmap to weight the surface by a data variable. The crashes layer used in this example has an attribute that tallies the number of drunk drivers involved in the incident. We can visualize where more drunk drivers are involved in fatal accidents by weighting by that field.
When a field is specified, each pixel's density is multiplied by the data value, so you need to adjust the max
accordingly.
const colors = ["rgba(115, 0, 115, 0)", "#820082", "#910091", "#a000a0", "#af00af", "#c300c3", "#d700d7", "#eb00eb", "#ff00ff", "#ff58a0", "#ff896b", "#ffb935", "#ffea00"];
layer.renderer = {
type: "heatmap",
field: "Number_of_Drinking_Drivers",
colorStops: [
{ color: colors[0], ratio: 0 },
{ color: colors[1], ratio: 0.083 },
{ color: colors[2], ratio: 0.166 },
{ color: colors[3], ratio: 0.249 },
{ color: colors[4], ratio: 0.332 },
{ color: colors[5], ratio: 0.415 },
{ color: colors[6], ratio: 0.498 },
{ color: colors[7], ratio: 0.581 },
{ color: colors[8], ratio: 0.664 },
{ color: colors[9], ratio: 0.747 },
{ color: colors[10], ratio: 0.83 },
{ color: colors[11], ratio: 0.913 },
{ color: colors[12], ratio: 1 }
],
radius: 18,
maxDensity: 0.00875,
minDensity: 0
};
Scale-dependent Heatmap
Dynamic heatmaps are only visually appropriate at a few scale levels. As the user zooms out, the heatmap will always appear hotter. As the user zooms in, the heatmap will appear colder. To prevent the user from viewing a heatmap that is too hot, you can set a minScale on the layer or as a view constraint.
As the user zooms in, the heatmap becomes irrelevant. Therefore, you should set a watch on the view to toggle the layer's heatmap to a simple renderer representing individual point locations.
const view = new MapView({
container: "viewDiv",
map: map,
constraints: {
minScale: 1155582,
snapToZoom: false
}
});
reactiveUtils.watch(
() => view.scale,
() => {
layer.renderer = view.scale <= 72224 ? simpleRenderer : heatmapRenderer;
}
);
Static heatmaps
Static heatmaps allow you to persist the visual density's of a heatmap from one scale across all scale levels. As the user zooms in and out, the heatmap will consistently show the same hot spots in the view.
As the user zooms in, the heatmap becomes irrelevant, so you may want to set a constraint on the MapView to prevent overzooming.
maxDensity: 0.319,
minDensity: 0,
// settings for heatmap apply only to this scale
// so renderer will look consistent without
// dynamically updating on zoom
referenceScale: 36111,
radius: 6,
legendOptions: {
minLabel: "Low tree density",
maxLabel: "High tree density"
}
};
Faded heatmaps
Configuring a heatmap renderer with proper density values can be difficult, especially when the starting view scale is unknown. The heatmap smart mapping renderer creator helps generate a suggested heatmap given the current view scale and background color.
It also automatically fades colors from dense areas (full opacity) to sparse areas (high transparency). The fading of colors helps establish a fuzzy boundary, which is often more appropriate than a hard edge for heatmap visualizations.
const { renderer } = await heatmapRendererCreator.createRenderer({ layer, view });
renderer.referenceScale = view.scale;
layer.renderer = renderer;
Related samples and resources
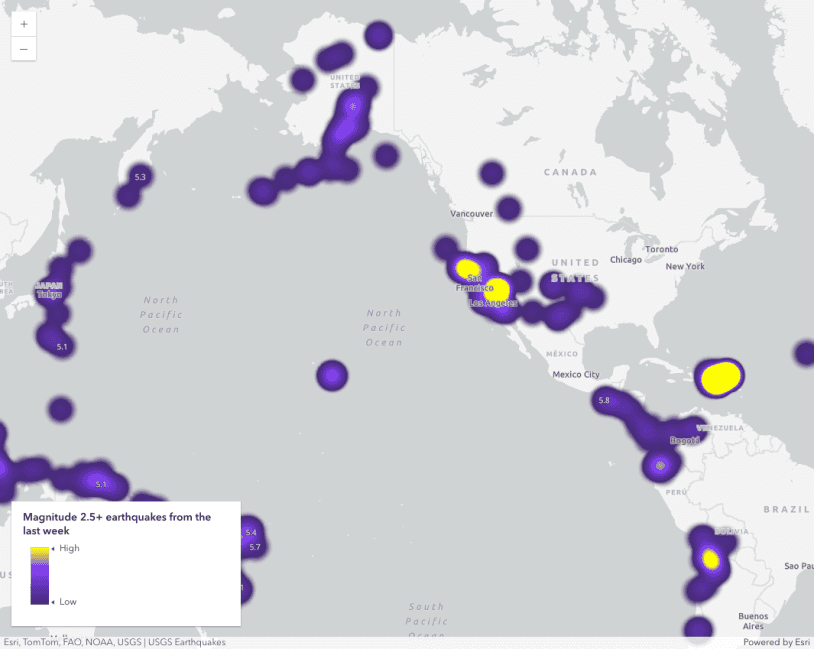
Intro to heatmap
Visualize points with a heatmap
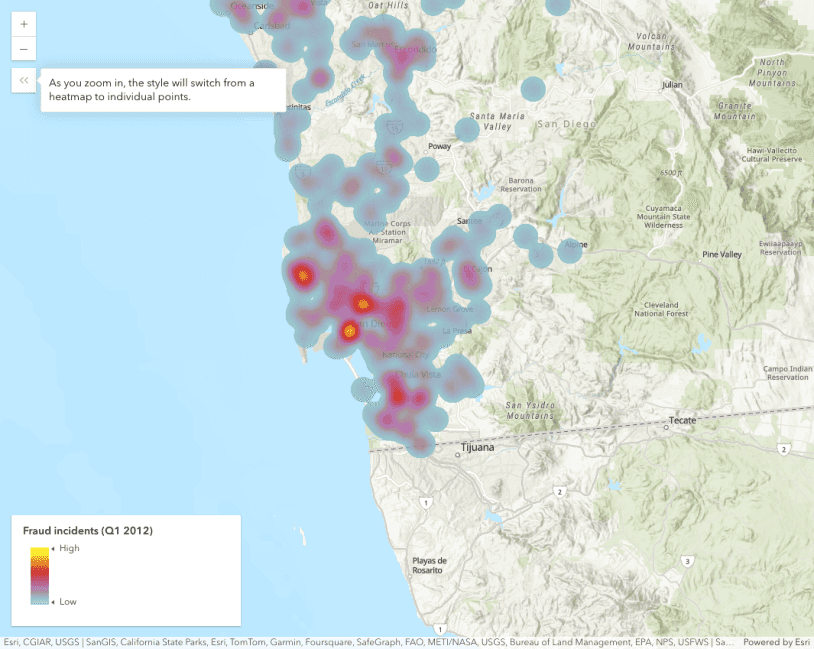
Create a scale-dependent visualization
Create a scale-dependent visualization
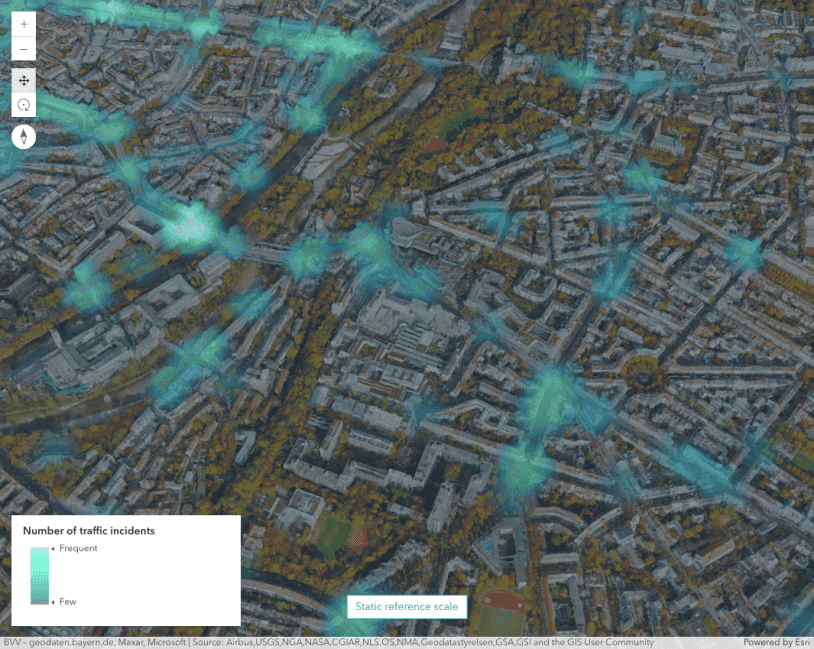
Visualize points in a scene with a heatmap
Visualize points in a scene with a heatmap
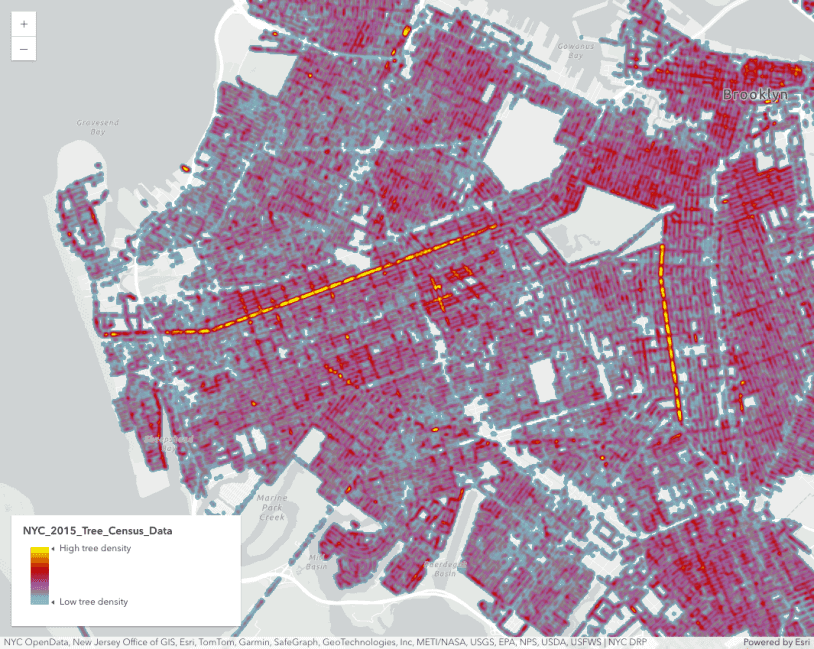
Create a static heatmap
Create a static heatmap that doesn't adjust based on scale
HeatmapRenderer
Read the Core API Reference for more information.
API support
The following table describes the geometry and view types that are suited well for each visualization technique.
2D | 3D | Points | Lines | Polygons | Mesh | Client-side | Server-side | |
---|---|---|---|---|---|---|---|---|
Clustering | ||||||||
Binning | ||||||||
Heatmap | ||||||||
Opacity | ||||||||
Bloom | ||||||||
Aggregation | ||||||||
Thinning | 1 | 1 | 1 | 2 | 3 | |||
Visible scale range |
- 1. Feature reduction selection not supported
- 2. Only by feature reduction selection
- 3. Only by scale-driven filter