This sample demonstrates how to query features represented by aggregate clusters. Clustering is a method of reducing features in a FeatureLayer, CSVLayer, GeoJSONLayer, WFSLayer, or OGCFeatureLayer by grouping them based on their spatial proximity to one another. Typically, clusters are proportionally sized based on the number of features within each cluster. See the Intro to clustering sample if you are unfamiliar with clustering.
Querying clustered features allows you to do the following:
- Query and display statistics describing the clustered features in the popup.
- Query the extent of the features and display it in the view.
- Query all features and calculate the convex hull.
To query a cluster's features, you must provide the ObjectID of the graphic representing the cluster to the Query.aggregateIds property and pass the parameters to the desired query method on the clustered layer's layer view.
const query = layerView.createQuery();
query.aggregateIds = [graphic.getObjectId()];
const { features } = await layerView.queryFeatures(query);
Query cluster statistics
To query statistics for the features included in a cluster, reference the ObjectID of the cluster graphic in Query.aggregate
, then specify the query outStatistics. Since the clustered layer has a UniqueValueRenderer, we'll use groupByFieldsForStatistics to group the statistics by the field used to categorize the features in the renderer.
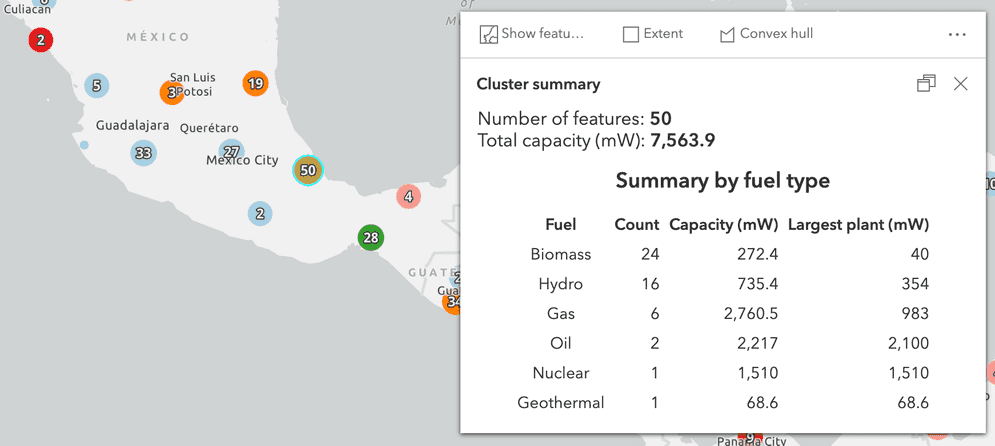
const query = layerView.createQuery();
query.aggregateIds = [graphic.getObjectId()];
query.groupByFieldsForStatistics = ["fuel1"];
query.outFields = ["capacity_mw", "fuel1"];
query.orderByFields = ["num_features desc"];
query.outStatistics = [
{
onStatisticField: "capacity_mw",
outStatisticFieldName: "capacity_total",
statisticType: "sum"
},
{
onStatisticField: "1",
outStatisticFieldName: "num_features",
statisticType: "count"
},
{
onStatisticField: "capacity_mw",
outStatisticFieldName: "capacity_max",
statisticType: "max"
}
];
const { features } = await layerView.queryFeatures(query);
const stats = features.map((feature) => feature.attributes);
Query cluster extent
You may want to display the extent of clustered features to the user. To do this, reference the cluster graphic's ObjectID in Query.aggregate
, and call the queryExtent method on the layer view.
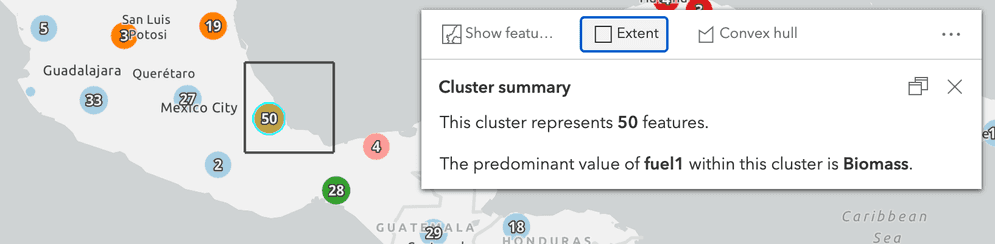
async function displayClusterExtent(graphic){
const query = layerView.createQuery();
query.aggregateIds = [ graphic.getObjectId() ];
const { extent } = await layerView.queryExtent(query);
const extentGraphic = {
geometry: extent,
symbol: {
type: "simple-fill",
outline: {
width: 1.5,
color: [ 75, 75, 75, 1 ]
},
style: "none",
color: [ 0, 0, 0, 0.1 ]
}
};
view.graphics.add(extentGraphic);
}
The cluster popup already displays the cluster extent by default when the browse features
action is clicked.
Display cluster convex hull
Perhaps it's better to show the convex hull of the clustered features rather than the extent since it more accurately represents their spatial distribution. The geometryEngine allows you to do that. Query for all the features in the cluster, get their geometries, then pass them to the convexHull method of geometryEngine.
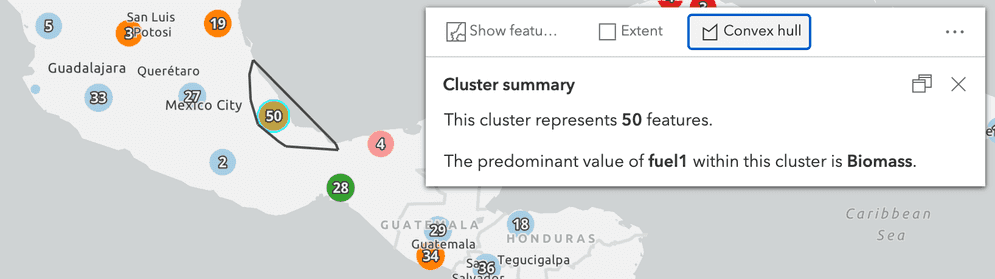
async function displayConvexHull(graphic) {
processParams(graphic, layerView);
const query = layerView.createQuery();
query.aggregateIds = [graphic.getObjectId()];
const { features } = await layerView.queryFeatures(query);
const geometries = features.map((feature) => feature.geometry);
const [convexHull] = geometryEngine.convexHull(geometries, true);
convexHullGraphic = new Graphic({
geometry: convexHull,
symbol: {
type: "simple-fill",
outline: {
width: 1.5,
color: [75, 75, 75, 1]
},
style: "none",
color: [0, 0, 0, 0.1]
}
});
view.graphics.add(convexHullGraphic);
}
Related samples and resources
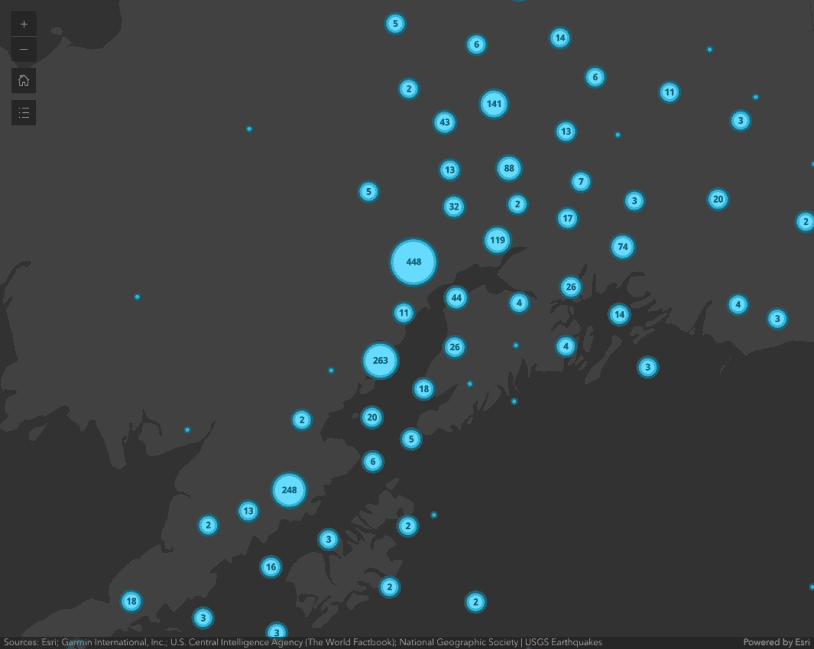
Intro to clustering
Intro to clustering
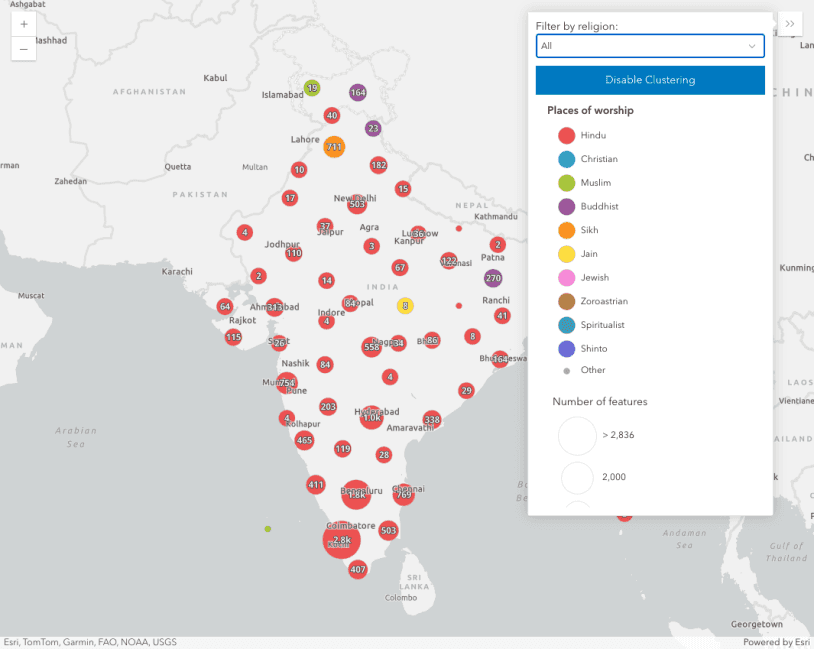
Clustering - generate suggested configuration
Clustering - generate suggested configuration
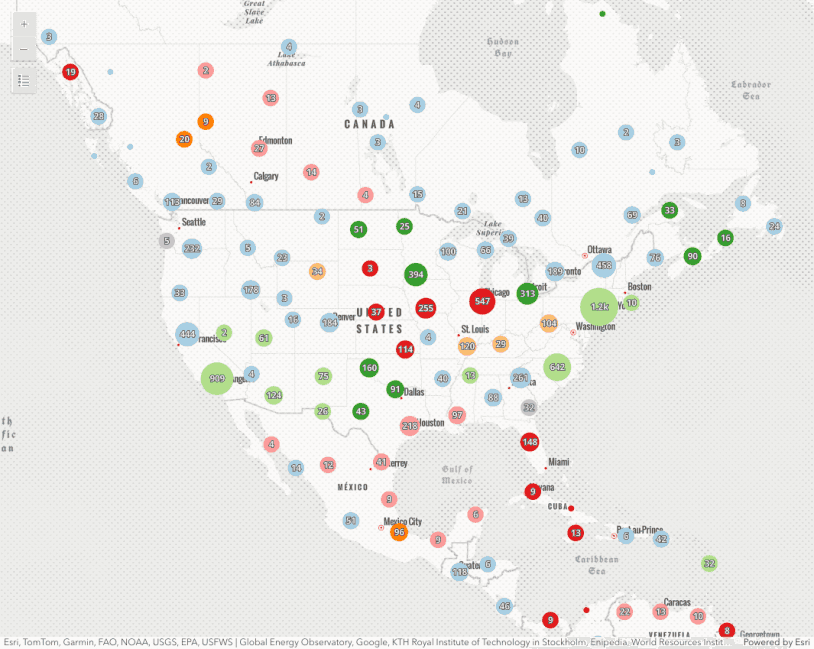
Clustering - filter popup features
This sample demonstrates how to filter clustered features within a cluster's popup.
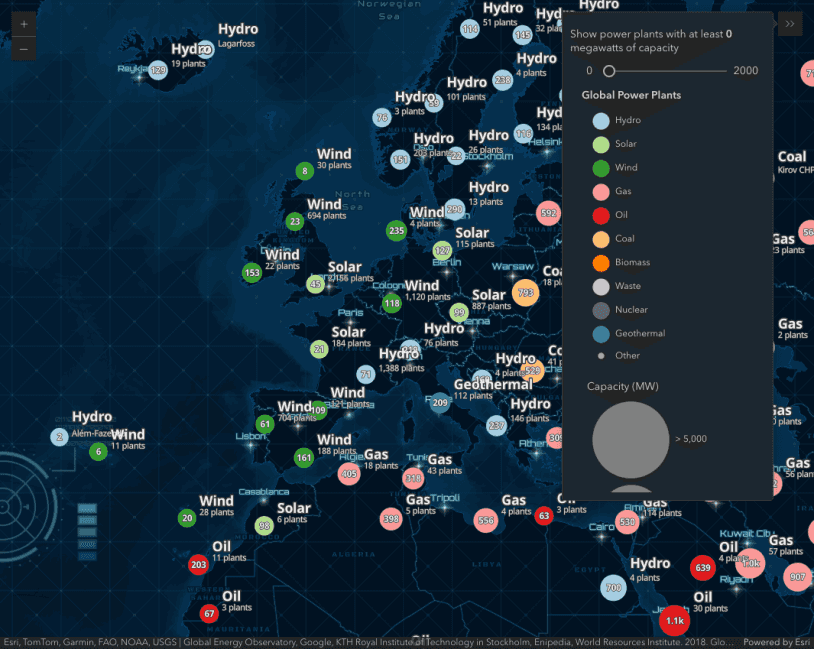
Clustering - advanced configuration
Clustering - advanced configuration
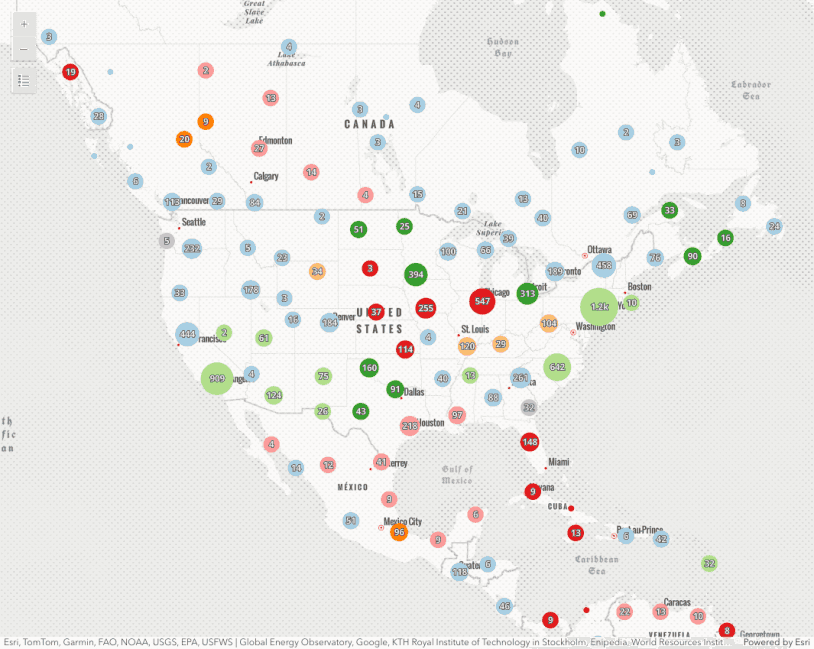
Popup charts for clusters
This sample demonstrates how to summarize clustered features using charts within a cluster's popup.
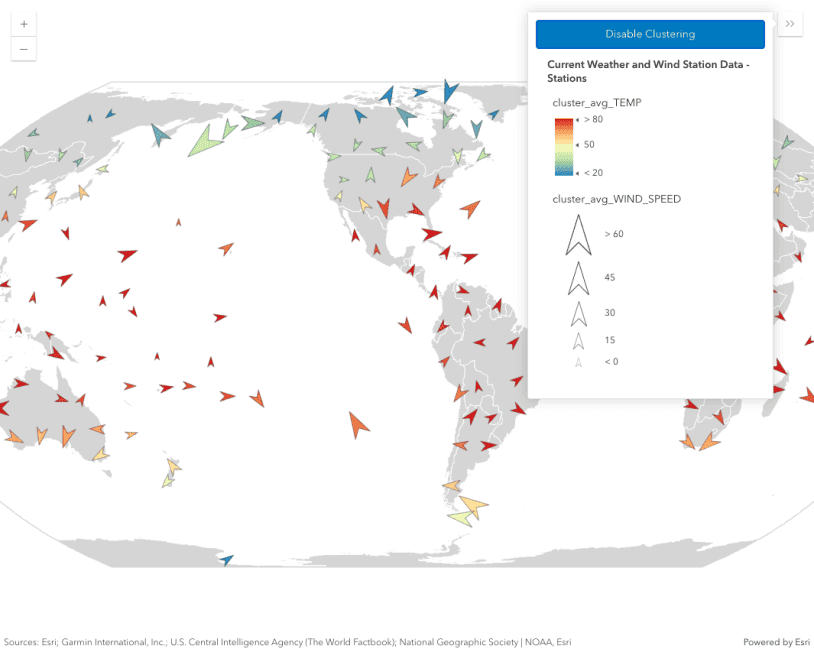
Clustering with visual variables
Clustering with visual variables
FeatureReductionCluster
Read the Core API Reference for more information.