What is Arcade?
Sometimes you need to render an attribute that doesn't exist in your layer. Arcade is an expression language that allows you to calculate values for each feature in a layer at runtime and use those values as the basis for a data-driven visualization. This is convenient when you need to derive new data values on data sources that update frequently, or on layers that you don't own.
See the Arcade - expression language guide for more details about how to use Arcade in other parts of the ArcGIS Maps SDK for JavaScript.
How Arcade works
Arcade expressions are referenced as strings in the value
property of ClassBreaksRenderer, UniqueValueRenderer, DotDensityRenderer or any visual variable: color, size, opacity, and rotation. When defined, it is always used instead of referencing a field
/normalization
.
When used in a ClassBreaksRenderer, DotDensityRenderer, or any of the visual variables, the value expression must evaluate to a number. Expressions may evaluate to either strings or numbers in UniqueValueRenderer.
Examples
Predominance with UniqueValueRenderer
In this example, Arcade is used to create a predominance map showing the most common political party affiliation in each U.S. county. The underlying layer has three fields that identify the number of republicans, democrats, and independent/non-party voters in each county. Since the service does not contain a field indicating the predominant party, we can write an Arcade expression to identify that for each feature.
The Arcade expression is referenced in the valueExpression property of a UniqueValueRenderer.
const renderer = {
type: "unique-value",
valueExpression: `
// store field values in variables with
// meaningful names. Each is the total count
// of votes for the respective party
var republican = $feature.MP06025a_B;
var democrat = $feature.MP06024a_B;
var independent = $feature.MP06026a_B;
var parties = [republican, democrat, independent];
// Match the maximum value with the label
// of the respective field and return it for
// use in a UniqueValueRenderer
return Decode( Max(parties),
republican, 'republican',
democrat, 'democrat',
independent, 'independent',
'n/a' );
`,
Then assign unique symbols for each expected return value in the renderer.
uniqueValueInfos: [
{
value: "democrat",
symbol: createSymbol("#00c3ff"),
label: "Democrat"
},
{
value: "republican",
symbol: createSymbol("#ff002e"),
label: "Republican"
},
{
value: "independent",
symbol: createSymbol("#faff00"),
label: "Independent/other party"
}
]
Arcade-driven opacity
Arcade can be used anywhere a data value is referenced in a renderer, including visual variables. The following snippet references an Arcade expression in an opacity visual variable to calculate the percentage of voters comprising the predominant party in each county. The opacity stops map the expected data values to opacity values.
Opaque counties indicate areas where the predominant political party comprises the vast majority of voters. Transparent counties show areas where there is a more diverse mix of individuals belonging to each political party.
renderer.visualVariables = [{
type: "opacity",
valueExpression: `
var republican = $feature.MP06025a_B;
var democrat = $feature.MP06024a_B;
var independent = $feature.MP06026a_B;
var parties = [republican, democrat, independent];
var total = Sum(parties);
var max = Max(parties);
return (max / total) * 100;
`,
valueExpressionTitle: "Share of registered voters comprising the dominant party",
stops: [
{ value: 33, opacity: 0.05, label: "< 33%" },
{ value: 44, opacity: 1.0, label: "> 44%" }
]
}];
See the Arcade - expression language guide for more details about how to use Arcade in other parts of the ArcGIS Maps SDK for JavaScript.
Related samples and resources
Arcade
Arcade allows you to dynamically calculate new data values for renderers, popups, and labels
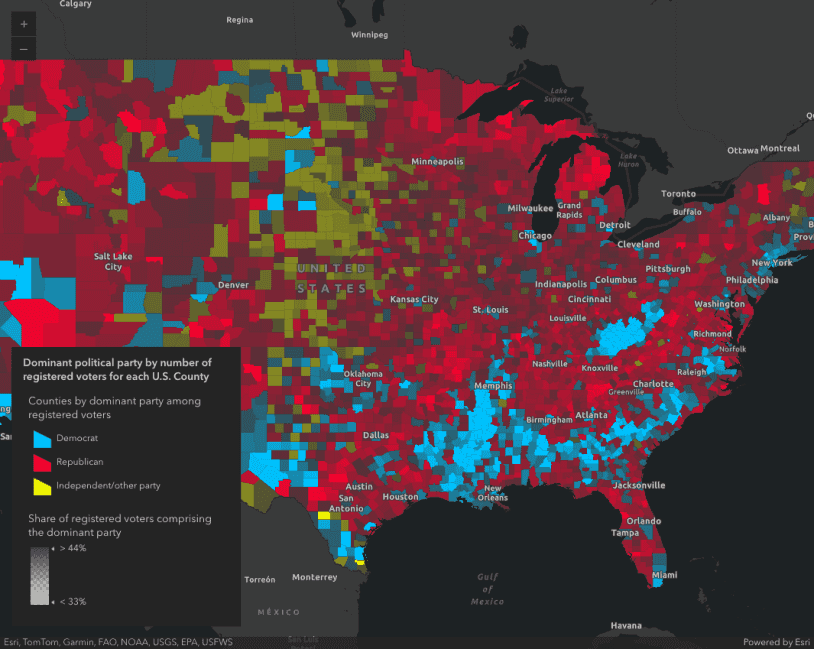
Create a custom visualization using Arcade
Create a custom visualization using Arcade
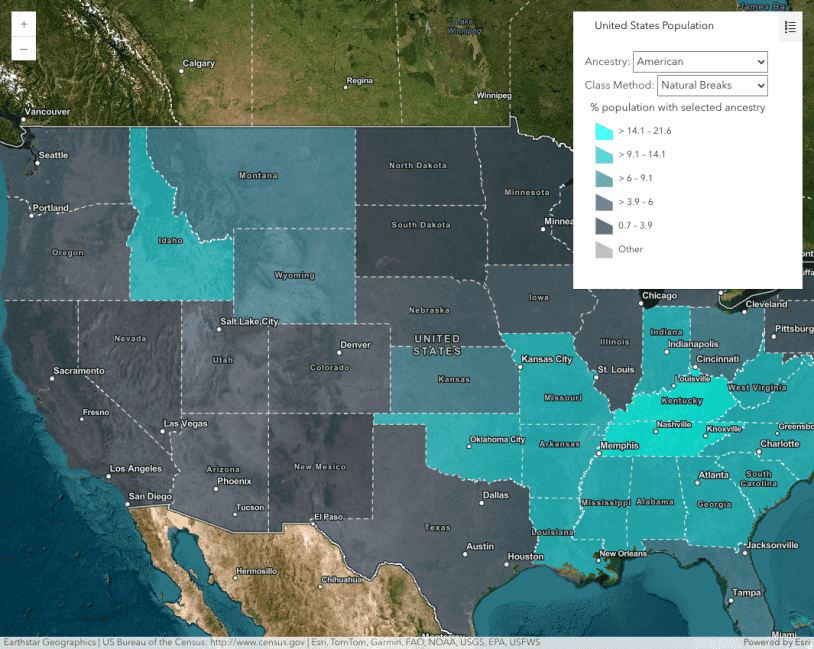
MapImageLayer - Explore data from a dynamic workspace
MapImageLayer - Explore data from a dynamic workspace
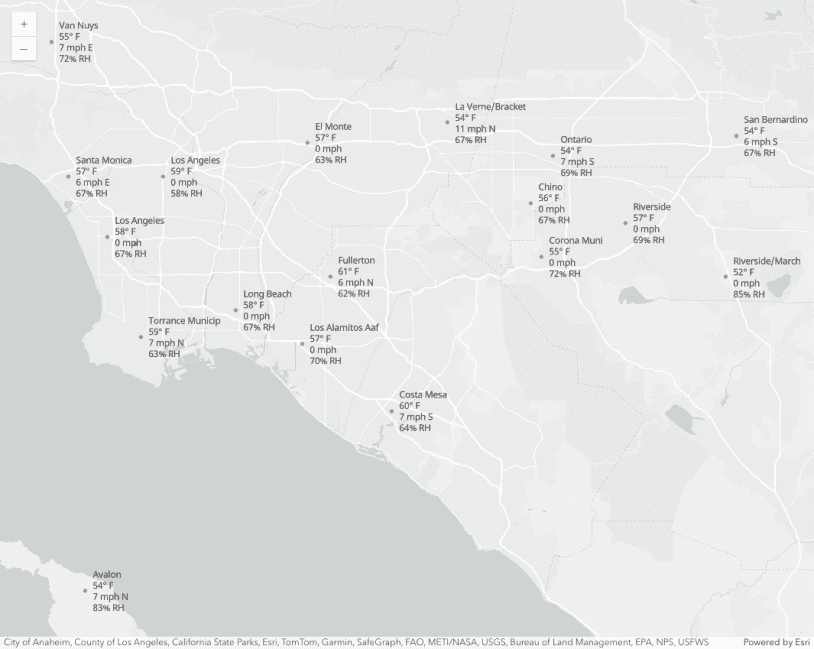
Multi-line labels
Multi-line labels
https://developers.arcgis.com/arcade